How to Send Emails Using a PHP Script
Spam or a flood of annoying emails is considered one of the biggest nuisances on the worldwide web. Spam messages bother server administrators because substantial spam may generate temporary system resource overload. It does not pose a serious threat even as spam brings inconvenience to users. However, a huge volume of outgoing spam can cause the anti-spam organization to backlist the mail IP producing problems in the delivery of messages.
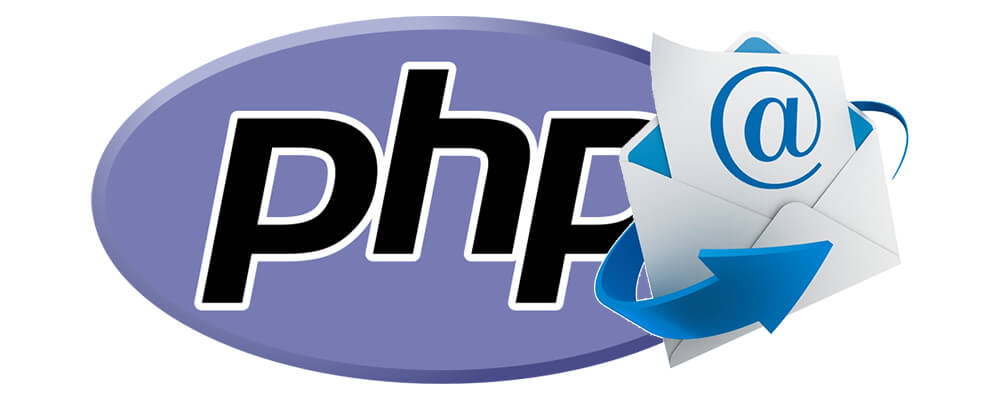
Jolt does not permit sending of emails without appropriate Simple Mail Transfer Protocol (SMTP) verification for these reasons.
- Spammers will not obtain your email passwords to send spam.
- Without STMP authentication, the script you upload may have several destination email accounts resulting in system resources overload and IP blacklisting.
- By sending emails without SMTP authentication, will be sent from username@server_hostname. Many large email providers will not accept an email from such an address and also consider it as spam.
You should employ SMTP authentication so your emails coming from PHP scripts will be considered clean and legitimate by all mail service providers. All our servers have proper PTR records and rDNS/DNS match).
Of course, many CMS solutions provide the option to use SMTP instead of the PHP mail () function (or wp_mail () function). In certain cases, you need to install an additional plugin or extension for this. If you have a self-written website or your CMS/local site builder does not support SMTP, Jolt offers an ideal solution.
The PHP mail () does not support SMTP authentication. It will not allow you to indicate your SMTP host, username, and password. However, you can use the PHP PEAR packages instead to deal with this issue. These are pre-installed for most PHP versions used on our server. Use the code below for instance:
<?php require_once "check_the_correct_path_below/Mail.php"; $from = "Sandra Sender <sender@example.com>"; $to = "Ramona Recipient <recipient@example.com>"; $subject = "Hi!"; $body = "Hi,\n\nHow are you?"; $host = "mail.example.com"; $username = "smtp_username"; $password = "smtp_password"; $headers = array ('From' => $from, 'To' => $to, 'Subject' => $subject); $smtp = Mail::factory('smtp', array ('host' => $host, 'auth' => true, 'username' => $username, 'password' => $password)); $mail = $smtp->send($to, $headers, $body); if (PEAR::isError($mail)) { echo("<p>" . $mail->getMessage() . "</p>"); } else { echo("<p>Message successfully sent!</p>"); } ?><br>
The path for the PEAR Mail.php library:
- For PHP native version (if you did not change the version in cPanel >> Select PHP Version) – /usr/local/lib/php/Mail.php
- For php 5.3 – /opt/alt/php53/usr/share/pear/Mail.php
- For php 5.4 – /opt/alt/php54/usr/share/pear/Mail.php
- For php 5.5 – /opt/alt/php55/usr/share/pear/Mail.php
- For php 5.6 – /opt/alt/php56/usr/share/pear/Mail.php
Please use localhost if your domain utilizes our mail server to process emails. For external mail servers, SMTP ports are closed by default on all our servers although we can open them for you. You can just contact us via Live Chat or HelpDesk for assistance. For a host, use the FQDN coming from your mail service provider.
You can also use the following code to allow SSL encryption in your emails:
<?php require_once "check_the_correct_path_above/Mail.php"; $from = "Sandra Sender <sender@example.com>"; $to = "Ramona Recipient <recipient@example.com>"; $subject = "Hi!"; $body = "Hi,\n\nHow are you?"; $host = "ssl://mail.example.com"; $port = "465"; $username = "smtp_username"; $password = "smtp_password"; $headers = array ('From' => $from, 'To' => $to, 'Subject' => $subject); $smtp = Mail::factory('smtp', array ('host' => $host, 'port' => $port, 'auth' => true, 'username' => $username, 'password' => $password)); $mail = $smtp->send($to, $headers, $body); if (PEAR::isError($mail)) { echo("<p>" . $mail->getMessage() . "</p>"); } else { echo("<p>Message successfully sent!</p>"); } ?><br>
In addition, if you have a VPS or Dedicated Server and opt for such rules (prohibiting mail without SMTP authentication), please contact us via HelpDesk for assistance as it requires a Managed Service for your server.
For additional questions, please contact us via Live Chat or HelpDesk.